An Introduction to the Command-Line (on Unix-like systems)
by Oliver; 201419. What is Scripting?
By this point, you should be comfortable using basic utilities like echo, cat, mkdir, cd, and ls. Let's enter a series of commands, creating a directory with an empty file inside it, for no particular reason:$ mkdir tmp $ cd tmp $ pwd /Users/oliver/tmp $ touch myfile.txt # the command touch creates an empty file $ ls myfile.txt $ ls myfile_2.txt # purposely execute a command we know will fail ls: cannot access myfile_2.txt: No such file or directoryWhat if we want to repeat the exact same sequence of commands 5 minutes later? Massive bombshell—we can save all of these commands in a file! And then run them whenever we like! Try this:
$ nano myscript.shand write the following:
# a first script
mkdir tmp
cd tmp
pwd
touch myfile.txt
ls
ls myfile_2.txt
Gratuitous screenshot:
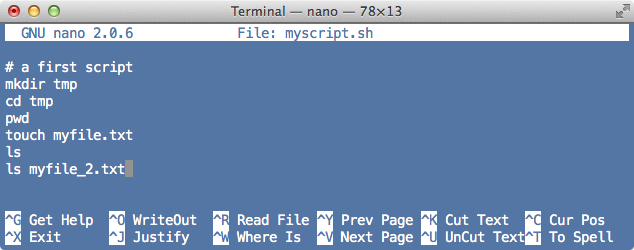
This file is called a script (.sh is a typical suffix for a shell script), and writing it constitutes our first step into the land of bona fide computer programming. In general usage, a script refers to a small program used to perform a niche task. What we've written is a recipe that says:
- create a directory called "tmp"
- go into that directory
- print our current path in the file system
- make a new file called "myfile.txt"
- list the contents of the directory we're in
- specifically list the file "myfile_2.txt" (which doesn't exist)
Let's run our program! Try:
$ ./myscript.sh -bash: ./myscript.sh: Permission deniedWTF! It's dysfunctional. What's going on here is that the file permissions are not set properly. In unix, when you create a file, the default permission is not executable. You can think of this as a brake that's been engaged and must be released before we can go (and do something potentially dangerous). First, let's look at the file permissions:
$ ls -hl myscript.sh -rw-r--r-- 1 oliver staff 75 Oct 12 11:43 myscript.shLet's change the permissions with the command chmod and execute the script:
$ chmod u+x myscript.sh # add executable(x) permission for the user(u) only
$ ls -hl myscript.sh
-rwxr--r-- 1 oliver staff 75 Oct 12 11:43 myscript.sh
$ ./myscript.sh /Users/oliver/tmp/tmp myfile.txt ls: cannot access myfile_2.txt: No such file or directoryNot bad. Did it work? Yes, it did because it's printed stuff out and we see it's created tmp/myfile.txt:
$ ls myfile.txt myscript.sh tmp $ ls tmp myfile.txtAn important note is that even though there was a cd in our script, if we type:
$ pwd /Users/oliver/tmpwe see that we're still in the same directory as we were in when we ran the script. Even though the script entered /Users/oliver/tmp/tmp, and did its bidding, we stay in /Users/oliver/tmp. Scripts always work this way—where they go is independent of where we go.
If you're wondering why anyone would write such a pointless script, you're right—it would be odd if we had occasion to repeat this combination of commands. There are some more realistic examples of scripting below.